Data structures|Data structures in python.|Lists and list operations python|What is a Data Structure in python
Introduction
What is a Data Structure?
Data Structures allows us to manage and organize the data in a way that it enables us to store collection of data, and perform operations on them according to our needs.
Some examples of Data structure in Python are:
- List
- Dictionary
- Tuples
- Set
These are known as implicit or built-in data structures.
Python also provides users to create their own Data Structures.
Example of such Data structures are:
- Queue
- Stack
- Tree ...etc
Let's Go through these data structures one by one.
1)Lists
Lists are used to store data of various data types like string,integers,..etc
in a sequential manner.Data type in the list is contained inside [ ] .In simple words a list is denoted by two square brackets[] and the data is stored inside it.
List is a type of Mutable Data type ,which means that the data stored inside it can be altered and several tasks can be performed on it.This is done using the Index values.
Index values-Index values are the unique addresses given to every element inside a list. We will understand the list by an example.
Example of a list:-a=["Pen","Pencil","Scale","Cups"]
So ,While going from Left to right the index value starts from 1 and gets incremented by 1 till the last element.
Therefore in the above example index values assigned to every element is as follows:-
INDEX VALUE ELEMENT
0 Pen
1 Pencil
2 Scale
3 Cups
While going from left to right the index value starts from -1 and gets substracted by 1 till the the first element(in this case "Pen").
INDEX VALUE ELEMENT
-4 Pen
-3 Pencil
-2 Scale
-1 Cups
Both system of indexing are correct and we can use them according to our ease.
Creating a list
To create a list we have to just create a variable and enter elements inside the square brackets.If their are no elements in the square bracket then the list is known as Empty list.
We will understand it with the help of Practical.
Operations on Lists:-
list.
append
():-Add an item to the end of the list.list.
extend
():-It is used to extend the list by appending all the items .list.
insert
():- It is used to Insert an item at a given position. list.
remove
(a):-It is used to Remove an item from the list whose value is equal to "a".- list.pop():-It is used to Remove the item at the given position in the list, and return it. If no index is specified list.pop() deletes the last element.
- list.clear():-It is used to Remove all items from the specified list.
- list.count(element):-It is used to Return the number of times element appears in the list.
- list.reverse():-It is used to Reverse the elements of the list.
We will understand it with the help of Practical:-
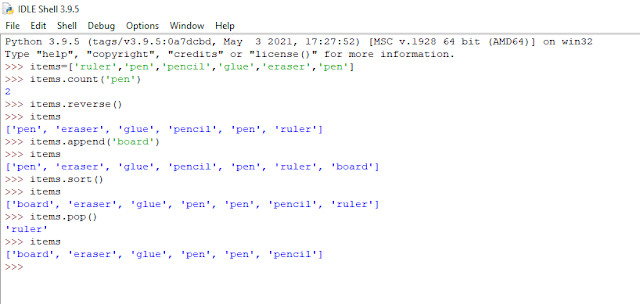
Click to view clear image
You can try all the possible operations of lists by yourself to enhance your knowledge.We can also access a particular element from the list using index values;for ex. print(items[index value])
list.
append
():-Add an item to the end of the list.list.
extend
():-It is used to extend the list by appending all the items .list.
insert
():- It is used to Insert an item at a given position. list.
remove
(a):-It is used to Remove an item from the list whose value is equal to "a".![]() |
Click to view clear image |
You can try all the possible operations of lists by yourself to enhance your knowledge.
How do we iterate List values using for loop:
Source Code:-
Output of the code will be :-
1
3
5
7
9
Detailed information on other data structures will be uploaded soon.
"Hope it helped"
Have anything in mind? Let me
know in the comment section👇
"Hope it helped"
Have anything in mind? Let me
know in the comment section👇
Have anything in mind? Let me
know in the comment section👇